Its weird because if i use the token manually i can request normally.
But when i try to use the same token for different requests i get the error:
That’s the function i’m using, straight from Postman (only did some minor tweaks)
const myHeaders = new Headers();
myHeaders.append("Authorization", `JWT ${token}`);
return fetch(query, {
method: "GET",
headers: myHeaders,
redirect: "follow",
})
.then((response) => response.text())
.then((result) => JSON.parse(result))
.catch((error) => console.error(error));
}
if i use the query as “https://api.baserow.io/api/database/tables/database/103959/” (103959 is my database id)
i get the tables just fine:
✓ Compiled /favicon.ico in 145ms (291 modules)
[
{ id: 281207, name: 'Customers', order: 1, database_id: 103959 },
{ id: 281208, name: 'Projects', order: 2, database_id: 103959 },
{ id: 286870, name: 'Campaings', order: 3, database_id: 103959 },
{ id: 286873, name: 'Sections', order: 4, database_id: 103959 }
]
then, i use the same token and same function to do another request
now using the query as “https://api.baserow.io/api/database/rows/table/${params.id}/” (params.id is my table id)
then i get the error:
{
detail: 'Access token is expired or invalid.',
error: 'ERROR_INVALID_ACCESS_TOKEN'
}
If i go to Postman and refresh the token, then use the new token manually, i can do both requests normally:
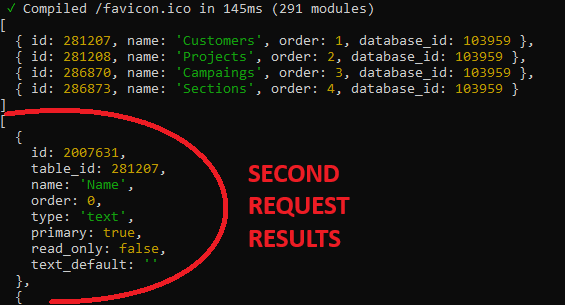
The only thing i changed between the two is the token, and since in the first test i could request the first after trying the second, i dont think the token expired
I really don’t know what else it could be.