I am developing an application in Appsmith and I want to send an image from the Camera widget to baserow.
I first tried through the upload-file endpoint, but this resulted in an error. Next I tried with the upload-via-url endpoint and passing a base64 string, but this also results in an error.
Is it possible to upload images from a base64 format?
Regards
Frederik
@frederikdc did you ever find a way to upload an image as base64? I can get binary files to upload, but not base64.
Not yet.
I followed your advice to check the Filepicker widget in Appsmith and I pass this data
{type: 'image/png', data: Camera1.imageRawBinary, name: 'takenbycamera.png'}
to the endpoint https://api.baserow.io/api/user-files/upload-file/.
I should try it out myself, but is it an option to use the atob() function that converts a base64 string into a binary file and upload this binary file?
@frederikdc I tried using the native JavaScript atob() function but the file wouldn’t open after it was uploaded to Baserow. Then I found this JS library to do the conversion and it worked!
Just import the library, then use Base64.atob()
to convert the string to binary. Then wrap that data
in a file object, with a type
, name
, and meta
property.
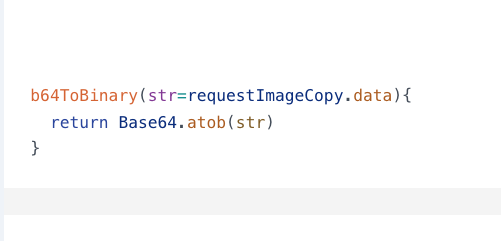
So here is how I got it to work because I could not get it to work with api endpoint.
This has a requirement that if you are using https on appsmith that you also have https on baserow. Also need to make a token with no permissions granted on it which is explained later.
The way it works is I use the browser fetch to send the blob from the camera to baserow and get the file information back. I then use that file information in the create or update call using the Api endpoint like normal. Then using the file information in the call back you can use the normal API calls. This can be a security risk since you have the token on the browser but the way I minimize that is I created a specific token that has no permissions. You can choose any workspace you want. This means it cannot read or write any data but it can still call other endpoints like upload file. I have not tested everything this token can do since we are using it on a closed system.
new Javascript object, I called mine “camOps”
export default {
uploadFileToBaserow: async (blobUrl, filename = "file") => {
const usingUrl = "https://{{ Your url base here }}/api/user-files/upload-file/"
const token = "{{ Special restricted token here }}"
// Get blob object from blobUrl
let blob = await fetch(blobUrl).then(r => r.blob());
// console.log(blob)
const typeSplit = blob.type.split('/')
let extension = 'webp'
if (typeSplit.length === 2) {
console.log('extension using', typeSplit[1])
extension = typeSplit[1]
}
const filename = `${filename}.${extension}`
const formData = new FormData()
formData.append('file', blob, filename)
const fileData = await fetch(usingUrl, {
method: 'post',
headers: {
Authorization: `Token ${token}`
},
body: formData
}).then((response) => response.json())
// console.log('response', returned)
return fileData
}
}
I use this like the following
export default {
submitForm: async () => {
// save picture if exists
const fileData = await camOps.uploadFileToBaserow(Camera1.imageBlobURL)
// console.log('file grabbed', fileData)
// Create record in baserow
const createRowData = {
note: "some note",
file: fileData
}
console.log('create data', createRowData)
const createdRow = await Create_Request.run(createRowData)
console.log('created request', createdRow)
}
}
Spent way too long on this figuring out something that works. The imitating the FilePicker structure worked that it sent a file but the file was never correct. This is the only way I could get the file in Baserow correctly.